소소한 개발 공부
[C#] checked / unchecked 본문
https://learn.microsoft.com/en-us/dotnet/csharp/language-reference/statements/checked-and-unchecked
checked and unchecked statements - control the overflow-checking context
The `checked` and `unchecked` statements control the overflow-checking context. In a check context, overflow causes an exception to be thrown. In an unchecked context, the result is truncated.
learn.microsoft.com
설명
checked와 unchecked 키워드는 정수 산술 연산 및 변환에 대해 오버플로 검사 컨텍스트를 지정하는 역할을 한다.
다시 말해,
checked로 지정한 컨텍스트는 오버플로와 언더플로 발생 시 에러를 발생시키고,
unchecked로 지정한 컨텍스트는 오버플로와 언더플로가 발생해도 무시하라는 의미이다.
코드
using System;
namespace ConsoleApplication1
{
internal class Program
{
public static void Main(string[] args)
{
uint a = uint.MaxValue;
unchecked
{
Console.WriteLine($"unchecked value : {a + 1}"); // 0
}
checked
{
Console.WriteLine($"checked value : {a + 1}"); // Unhandled Exception - overflow
}
}
}
}
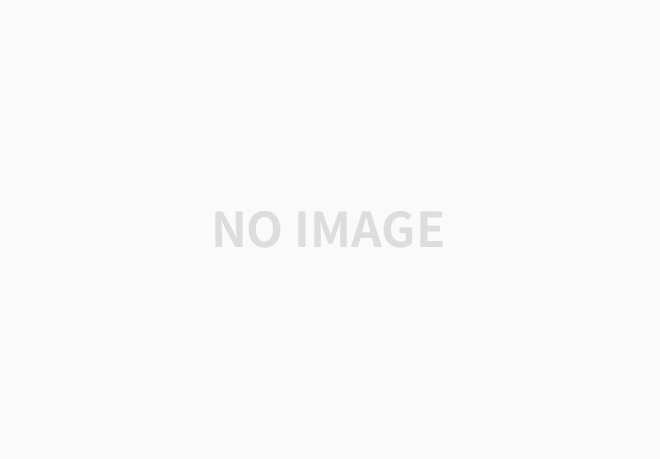
uint는 unsigned int로 0 ~ 4,294,967,295 사이 값을 나타낸다.
오버플로가 발생하는 경우(예를 들어, max + 1) 0부터 다시 시작한다.
위의 unchecked 예시에서는 MaxValue + 1 값을 출력하는데, 이때 오버플로에 대한 에러를 무시하므로 0을 출력한다.
반면에 checked 예시에서는 오버플로를 걸러줘 OverflowException이 걸리게 된다.
checked, unchecked를 쓰지 않은 경우
uint a = uint.MaxValue;
Console.WriteLine($"value : {a + 1}"); // 0
컨텍스트를 지정해주지 않고 오버플로를 발생시키는 경우 unchecked 컨텍스트를 지정한 것과 같은 결과를 낸다.
이는 try-catch문으로 예외처리를 해도 같은 결과를 낸다.
public static void Main(string[] args)
{
uint a = uint.MaxValue;
try
{
Console.WriteLine($"value : {a + 1}"); // 0
}
catch (Exception e)
{
Console.WriteLine(e);
throw;
}
}
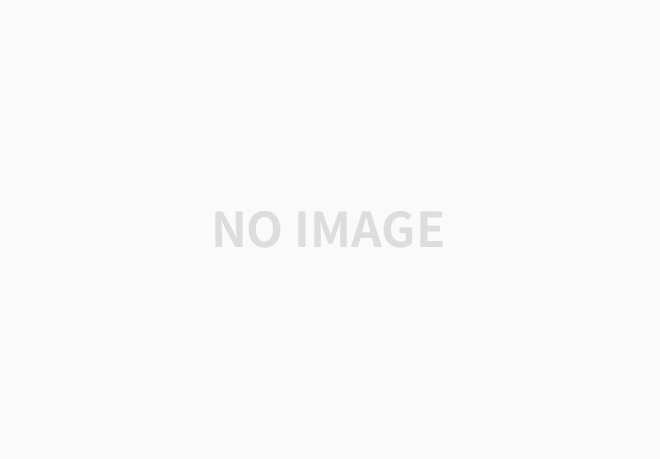
'프로그래밍 > C#' 카테고리의 다른 글
[C#] 진법에 따른 숫자 리터럴 (0) | 2023.01.26 |
---|---|
[C#] stopwatch 시간 측정 (0) | 2023.01.25 |
[C#] @의 의미 (0) | 2023.01.18 |
[C#] 입출력 Console.Write, Console.Read (0) | 2022.12.20 |
[C#] Switch (0) | 2022.12.20 |